Agent Actions
What's an Action?
An Action in the Snak is a task that an agent can perform. Actions are implemented as tools with defined schemas and execution logic. Each action represents a specific capability, like transferring tokens or checking balances. These tools are static and implemented by developers to ensure desired and secure Agent behavior.
Storage and Implementation
Tool Registry System
Actions are stored and managed through a central registry system in agents/src/tools.ts
. Each action is registered as a tool with:
name
: A unique nameplugin
: the name of the plugindescription
: The tool description for the model to understand it's behaviorschema
: Input schema (using Zod) for the model to understand which parameters it should extract from the requestexecute
: The function that will be executed by LangChain
// Example tool registration
StarknetToolRegistry.registerTool({
name: "transfer",
plugin : "token"
description: "Transfer ERC20 tokens to a specific address",
schema: Transferschema,
execute: transfer,
});
Plugin Architecture
Each plugin has its own directory under ./plugins/
with a standardized
structure for organization.
/plugins/
└── [plugin-name]/
├── src/
│ ├── abis/ # Plugin-specific ABIs
│ ├── actions/ # Plugin actions
│ ├── tools/ # Plugin tools
│ ├── schema/ # Plugin schema
│ ├── utils/ # Utility functions
│ ├── constant/ # Constants and addresses
│ ├── interface/ # Interfaces and schemas
│ ├── types/ # Type definitions
│ └── index.ts
├── test/
├── package.json
├── tsconfig.json
├── tsconfig.build.json
└── readme.md
Interacting with Actions
There are currently four ways to run actions through your agents:
The kit is available as an NPM package that you can import in your projects to leverage agent actions.
Install Package
npm install starknet-agent-kit
Choose Integration Method
You can integrate the kit using either the Agent interface or individual tools:
Option A: Agent Interface
import { StarknetAgent } from "starknet-agent-kit";
const agent = new StarknetAgent({
aiProviderApiKey: "your-key",
aiModel: "claude-3-5-sonnet-latest",
provider: rpcProvider,
accountPublicKey: "your-public-key",
accountPrivateKey: "your-private-key",
});
// Execute actions using natural language
await agent.execute("Transfer 5 USDC to 0x123...");
Option B: Individual Tools
import { getBalance, transfer, swapTokens } from "starknet-agent-kit";
// Use tools individually
const balance = await getBalance(address);
const transferResult = await transfer(recipient, amount);
Available Actions Catalog
Explore plugins
You can explore the implemented plugins and their available actions directly in the source code under ./plugins
(opens in a new tab) or through our high-level plugin interface.
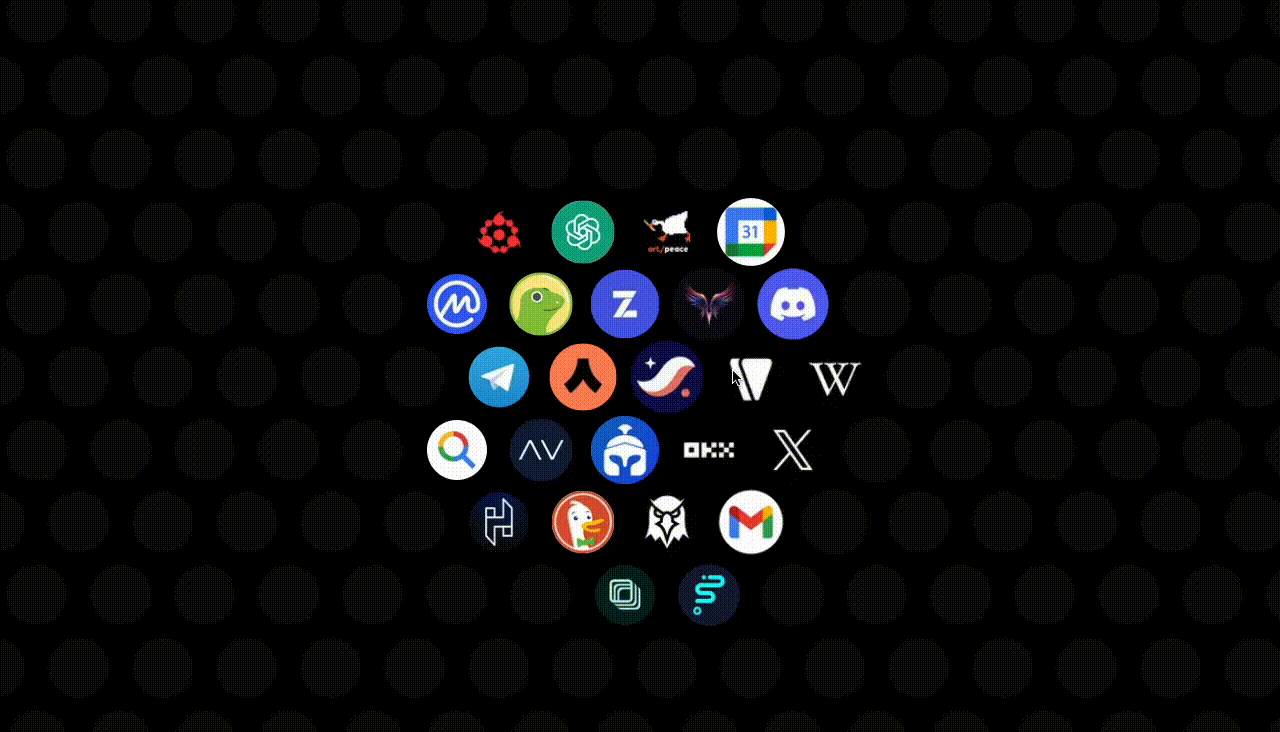
You can explore our complete catalog of plugins and their associated actions on Starkagent (opens in a new tab), where detailed documentation is available for each integration.
Extended Capabilities
To add action capabilities, you can either leverage LangChain tools or add your own custom Plugins and Actions.