Integrate LangChain Tools
LangChain tools can significantly enhance your Starknet agent's capabilities by providing access to a wide range of functionalities beyond blockchain interactions.
Overview
LangChain provides a rich ecosystem of tools that enable your agent to:
- Access external APIs and data sources
- Process and analyze information
- Interact with various services
- Handle different types of media and content
Getting Started
Install Dependencies
First, add the necessary LangChain packages to your project:
pnpm add langchain @langchain/core @langchain/community
Create a Tool Wrapper
Create a wrapper to adapt LangChain tools to the Snak format:
// ./plugins/langchain/tools.ts
import { Tool } from "langchain/tools";
import { z } from "zod";
import { StarknetToolRegistry } from "../../registry";
export class LangChainToolWrapper {
private tool: Tool;
constructor(tool: Tool) {
this.tool = tool;
}
registerAsTool() {
const schema = z.object({
input: z.string().describe(this.tool.description),
});
StarknetToolRegistry.registerTool({
name: this.tool.name,
description: this.tool.description,
schema: schema,
execute: async (agent, params) => {
try {
const result = await this.tool.call(params.input);
return JSON.stringify({ status: "success", data: result });
} catch (error) {
return JSON.stringify({
status: "error",
error: error instanceof Error ? error.message : "Unknown error",
});
}
},
});
}
}
Register Tools
Add your LangChain tools to your agent configuration:
// config/agent/index.ts
import { WikipediaQueryRun } from "langchain/tools";
import { LangChainToolWrapper } from ".././plugins/langchain/tools";
const wikipediaTool = new WikipediaQueryRun({
topKResults: 3,
maxDocumentLength: 4000,
});
new LangChainToolWrapper(wikipediaTool).registerAsTool();
Available Tools
Explore plugins
You can explore the implemented LangChain tools and their available actions directly in the source code under ./plugins
(opens in a new tab) or through our high-level plugin interface.
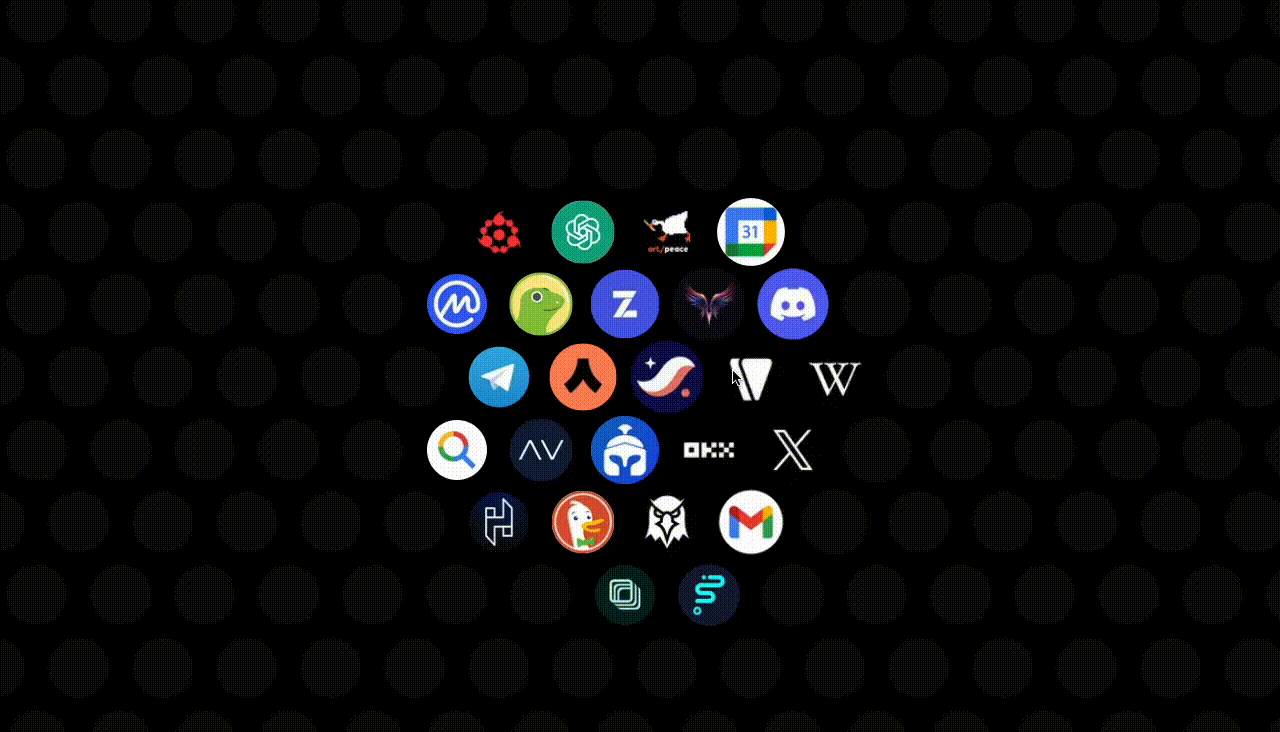